Connect with Python
Prerequisite: Install the Agent onto your machine using the curl command
Send a message
Let's have your robot send its first message to the cloud! We'll start by sending a GPS position from your robot. Create a file called robot.py
and copy in the code below.
This code sample sends latitude and longitude data of type sensor_msgs/NavSatFix
to the /location
topic.
from freedomrobotics.link import Link
# Connect to the cloud with Link
# you can instantiate multiple Link objects by using different names (core, my_node1)
freedom = Link("core")
# Send your GPS position
freedom.message("/location", \
"sensor_msgs/NavSatFix", \
{"latitude": 37.778454,"longitude": -122.389171})
Next, run your code with python robot.py
. The message will show up in the Freedom App in your STREAM dashboard, showing a map with the location of your device.
In addition to the freedom.message
method for sending data, the Python module has a freedom.log
method for logging and a freedom.image
method for sending images. You can read more in the Python module reference.
Receive a message
Let's now send a simple command to your robot.
Set up a callback
To prepare your robot to receive and respond to a command, we'll need to set up a callback. Create a file called callback.py
and copy in the code below.
from time import sleep
from freedomrobotics.link import Link
keep_alive = True
# Create a callback to handle remote messages
def callback(msg):
global keep_alive
freedom.log("info", "I heard " + str(msg) )
if msg["topic"] == "/commands":
if msg["message"]["data"] == "mission":
freedom.log("info", "Running mission...")
elif msg["message"]["data"] == "shutdown":
freedom.log("info", "Shutting down...")
keep_alive = False
# Connect to the cloud with Link
freedom = Link(name="Core", command_callback=callback)
# Run until told to shut down
while keep_alive:
sleep(1)
Run the code with python callback.py
. Your robot is now waiting patiently for a remote command.
Test the callback
To check that the callback works, we'll send a command to your device using Freedom's REST API. We can create a login token and use that for the Freedom Rest API calls.
- For this exercise, create a token you using Freedom's REST API Endpoint. We will want to ensure
put
is enabled for accounts, device, device_commands.
{
"token": "",
"allowed_actions": {
"device": ["put"],
"device_data": ["put"],
"device_commands": ["put"],
"devices": ["put"],
"account": ["put"]
},
"accounts": ["{{account_id}}"],
"devices": "*"
}
Next, in your computer's file system, create a file on your machine called test.sh.
touch simple_device_command.sh
- Edit the file with the example curl command below. The variables will be replaced with the token you have created using the API, your respective account id, and the device you would like to target.
Your account and device id can be located in your file system at ~/.config/freedomrobotics/credentials
.
TOKEN="<<USER_TOKEN>>"
SECRET="<<USER_SECRET>>"
ACCOUNT="<<ACCOUNT>>"
DEVICE="<<DEVICE>>"
HEADERS="-H content-type:application/json -H mc_token:$TOKEN -H mc_secret:$SECRET"
DATA="[{
\"platform\": \"custom\",
\"utc_time\": `date +%s`,
\"topic\": \"/commands\",
\"type\": \"std_msgs/String\",
\"expiration_secs\": 60,
\"message\": {\"data\":\"mission\"}
}]"
curl -v $HEADERS -d "$DATA" -X PUT "<<API_URL>>accounts/$ACCOUNT/devices/$DEVICE/commands"
- Run the file using bash.
bash simple_device_command.sh
The message will be received in the callback you set up. Once your robot receives the command, it will log Running mission...
.
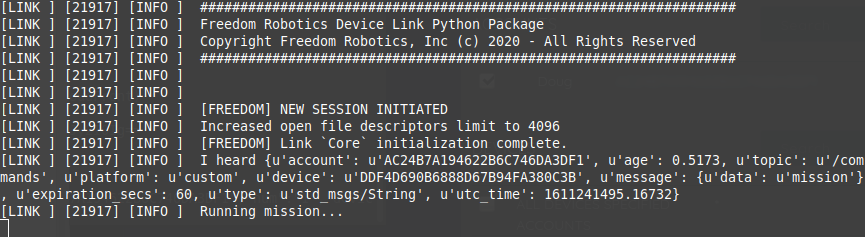
Change mission
to shutdown
and send a new command. Your application will now shut down remotely.
Success! You now know how to use Freedom to upload data and communicate with your robot through the cloud.
What's next?
If you would like to read walkthroughs of usage for key aspects of the system, the guides for Monitoring, Alerts and Integrations, and Teleop and Control are a great place to start.
If you prefer reviewing detailed API specs and references, you can do that also.
If you like to poke around applications, go to the Freedom App and explore!
Updated about 3 years ago